캡슈컴포넌트는 충돌감지에 사용된다
폴리곤에 직접 충돌감지를 할 수 있지만 복잡한 폴리곤의 경우 너무 많은 리소스비용이든다.
이를 방지하기위해 캡슐컴포넌트를 사용한다.
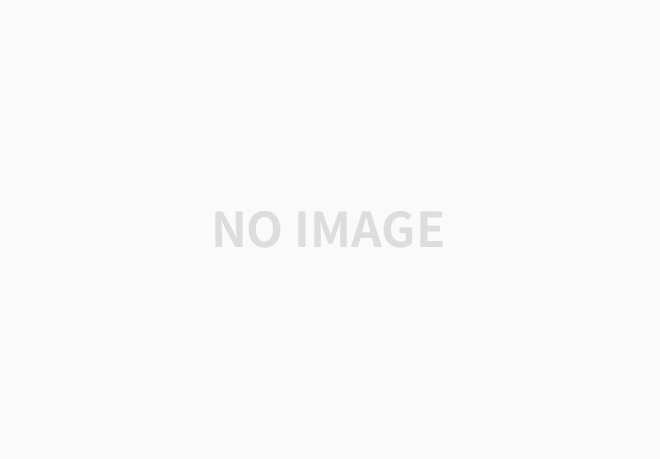
1. 새로운 pawn의 생성
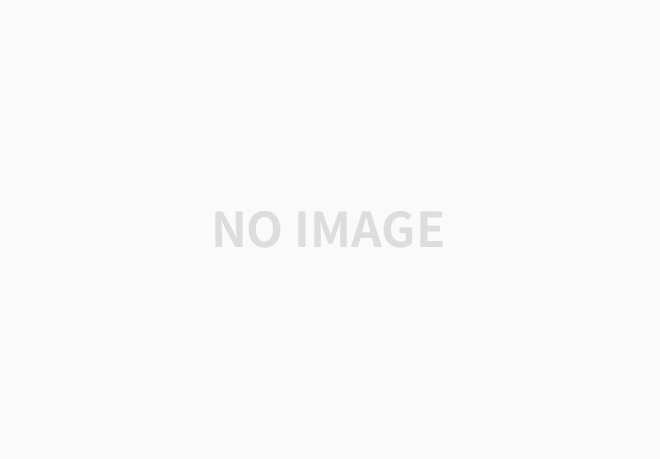
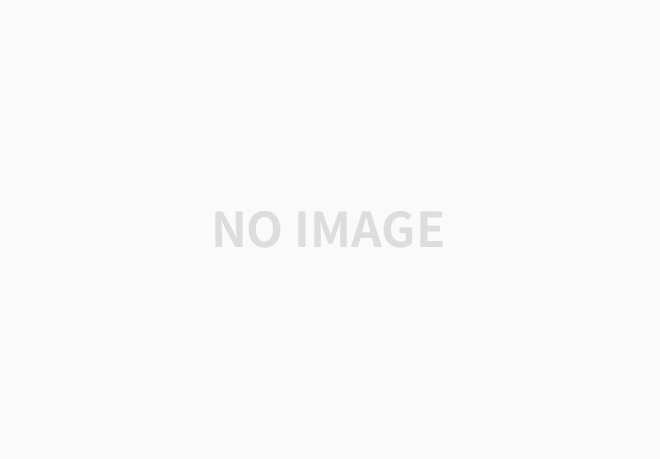
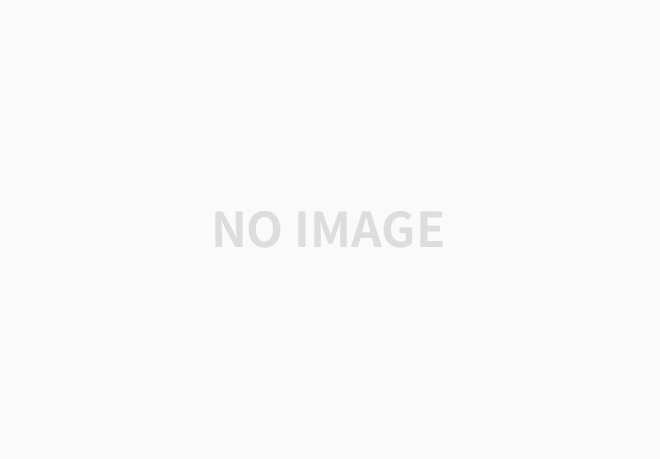
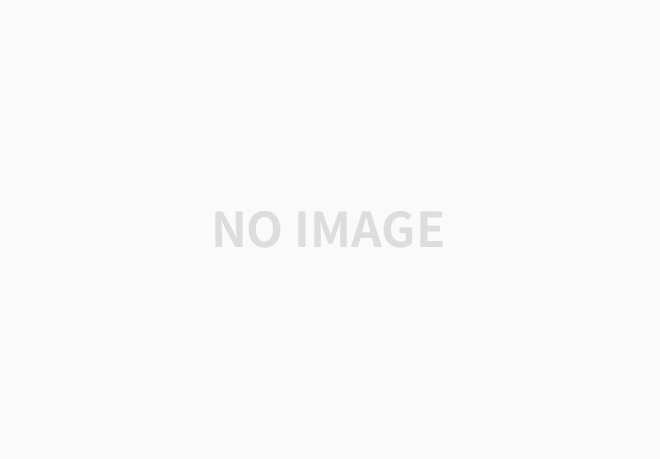
# Bird.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Pawn.h"
#include "Components/CapsuleComponent.h"
#include "Bird.generated.h"
UCLASS()
class TEST01_API ABird : public APawn
{
GENERATED_BODY()
public:
ABird();
virtual void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
virtual void Tick(float DeltaTime) override;
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
private:
// 캡슐의 변수 선언
UPROPERTY(VisibleAnywhere)
UCapsuleComponent* Capsule;
};
# Bird.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "Bird.h"
ABird::ABird()
{
PrimaryActorTick.bCanEverTick = true;
//생성자 위치에서 Capsule의 변수를 설정해줌
Capsule = CreateDefaultSubobject<UCapsuleComponent>(TEXT("Capsule"));
Capsule->SetCapsuleHalfHeight(20.f);
Capsule->SetCapsuleRadius(15.f);
// 폰의 최상위 컴포넌트를 Capsule로 바꿔준다.
SetRootComponent(Capsule);
}
void ABird::BeginPlay()
{
Super::BeginPlay();
}
void ABird::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
void ABird::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
}
# 블루프린트


Include Header
위의 예제에서 우리는 UCapsuleComponent를 Bird.h에 include 했다.
이와 같은 방식은 문제가 있다.
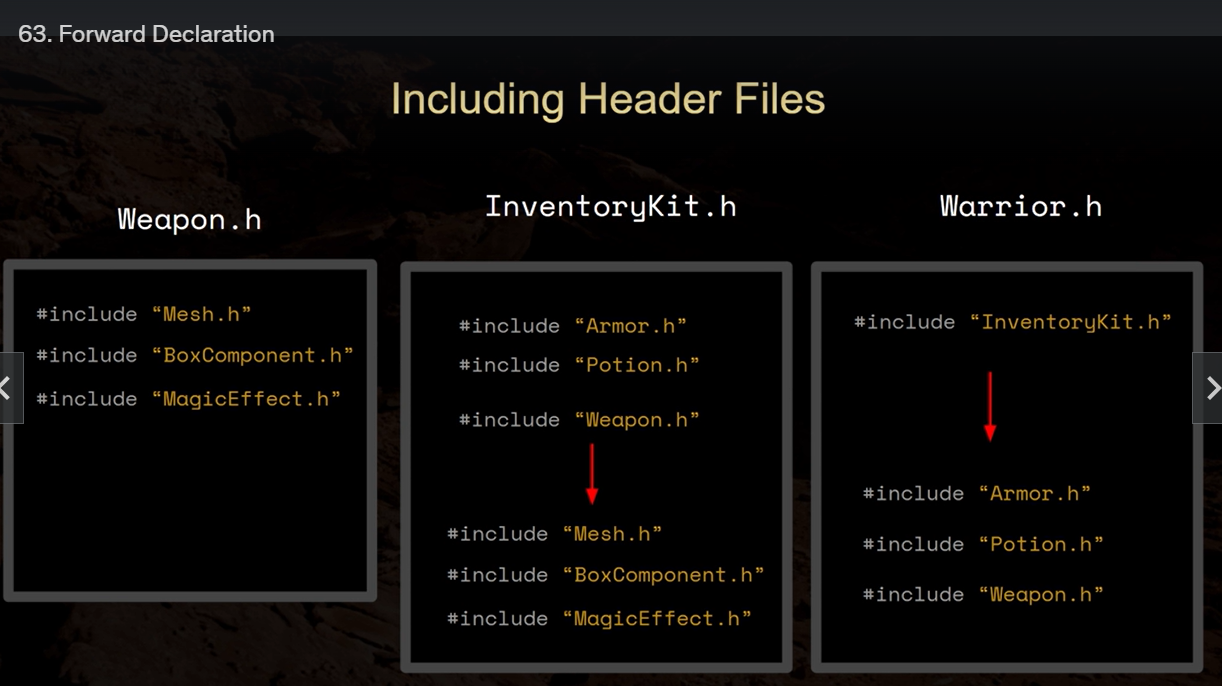
이와 같이 최종적으로 사용하는 파일의 .h 파일은 기하급수적으로 많은 헤더 파일을 include 하게 될 뿐만아니라.

각 헤더파일끼리 Cross 참조하게 되는 경우 에러가 발생 한다.
이를 해결하기위해 UCapsuleComonent가 Class임을 명시하고 cpp 파일에서 include를 받는것이 바람직하다.
변경된 Bird.h 파일
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Pawn.h"
// UCapsuleComonent의 include가 사라졋다.
#include "Bird.generated.h"
UCLASS()
class TEST01_API ABird : public APawn
{
GENERATED_BODY()
public:
ABird();
virtual void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
virtual void Tick(float DeltaTime) override;
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
private:
// 캡슐의 변수 선언 해당 변수가 class임을 명시한다.
UPROPERTY(VisibleAnywhere)
class UCapsuleComponent* Capsule;
};
Bird.cpp
// 컴포넌트 include
#include "Components/CapsuleComponent.h"
#include "Bird.h"
ABird::ABird()
{
PrimaryActorTick.bCanEverTick = true;
//생성자 위치에서 Capsule의 변수를 설정해줌
Capsule = CreateDefaultSubobject<UCapsuleComponent>(TEXT("Capsule"));
Capsule->SetCapsuleHalfHeight(20.f);
Capsule->SetCapsuleRadius(15.f);
// 폰의 최상위 컴포넌트를 Capsule로 바꿔준다.
SetRootComponent(Capsule);
}
// 이하 생략
'기타 > UnReal Engine' 카테고리의 다른 글
Skeletal Mesh Component (0) | 2023.12.03 |
---|---|
함수의 노출 (UFUNCTION, templateFunction) (0) | 2023.11.21 |
멤버변수의 노출(UPROPERTY) (0) | 2023.11.21 |
멤버변수의 추가와 sin함수 (1) | 2023.11.21 |
오브젝트의 이동 (초당 이동거리, Persistent, AddActorWorldOffser) (0) | 2023.11.21 |